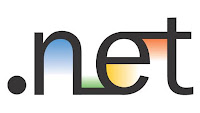
This post has reference to part one of this Article.
Create a new Class with the name “MyLinq”. We are going to use the DataContextFactory Class which we created in Part One. Also assume in our database we have Table with name “User” and with the following Definition.
Also inside the “Linq to Sql” Template, the name of the “User” Table interface is “UserEntity”. Now lets see how we can INSERT or UPDATE Data Rows in the User Table. Use the following block of code within MyLinq class,
using (DataDataContext db = DataContextFactory.CreateContext())
InsertUpdateUser(db, userName, phoneNumber);
db.SubmitChanges();
}
}
{
try
{
var userentity = (from entity in db.UserEntities
where entity.UserName == userName
select entity)
List<UserEntity> uE = userentity.ToList();
if (uE.Count == 0)
{
UserEntity userE = new UserEntity();
userE.UserName = userName;
userE.PhoneNumber = phonenumber;
db.UserEntities.InsertOnSubmit(userE);
}
else
{
userE.PhoneNumber = phonenumber;
}
}
catch(Exception)
{
throw new Exception(“Unable to Insert or Update the Database”);
}
}
Now use the below Two methods to DELETE option,
{
using (DataDataContext db = DataContextFactory.CreateContext())
{
DeleteUser(db, userName);
db.SubmitChanges();
}
}
{
try
{
db.UserEntities.DeleteAllOnSubmit(from entity in db.UserEntities
where entity.UserName == userName
select entity);
}
catch (Exception)
{
throw new Exception(“Unable to Delete”);
}
}